Hi everyone,
I’m working on a project using the STM32F103RBT6 to interface with the TUSS4470. However, I’ve encountered several issues during the initialization process:
- Unexpected Reset Values: The register values I read after powering on do not match the reset values specified in the TUSS4470 datasheet.
- Write/Read Inconsistency: When I try to write to a register and read it back, the value does not update as expected.
- Abnormal SDO Feedback: After writing to a register, the feedback on the SDO line appears incorrect.
Additional Notes:
- I’m using a custom-designed circuit board that I assembled using SMT (surface mount technology). I’ve tested two separate PCBs, and both show the same issues.
- My development environment is IAR Embedded Workbench 8.32.1.
Has anyone encountered similar issues with the TUSS4470? I would appreciate any advice on debugging or identifying potential causes.
Below are the code snippet and snipaste I am using for initialization and SPI communication.
#include "TUSS4470.h" uint16_t init_check_buffer[13] = {0}; uint16_t write_back[13] = {0}; static uint8_t calculateParity(uint8_t txbuffer[]); void TUSS4470_init() { TUSS4470_Write_Register(BPF_CONFIG_1, 0x0, &write_back[0]); TUSS4470_Write_Register(BPF_CONFIG_2, 0x0, &write_back[1]); TUSS4470_Write_Register(DEV_CTRL_1, 0x0, &write_back[2]); TUSS4470_Write_Register(DEV_CTRL_2, 0x0, &write_back[3]); TUSS4470_Write_Register(DEV_CTRL_3, 0x0, &write_back[4]); TUSS4470_Write_Register(VDRV_CTRL, 0x20, &write_back[5]); TUSS4470_Write_Register(ECHO_INT_CONFIG, 0x7, &write_back[6]); TUSS4470_Write_Register(ZC_CONFIG, 0x14, &write_back[7]); TUSS4470_Write_Register(BURST_PULSE, 0x1, &write_back[8]); TUSS4470_Write_Register(TOF_CONFIG, 0x0, &write_back[9]); TUSS4470_Write_Register(DEV_STAT, 0x0, &write_back[10]); TUSS4470_Write_Register(DEVICE_ID, 0xB9, &write_back[11]); TUSS4470_Write_Register(REV_ID, 0x2, &write_back[12]); HAL_Delay(500); TUSS4470_Read_Register(BPF_CONFIG_1, &init_check_buffer[0]); TUSS4470_Read_Register(BPF_CONFIG_2, &init_check_buffer[1]); TUSS4470_Read_Register(DEV_CTRL_1, &init_check_buffer[2]); TUSS4470_Read_Register(DEV_CTRL_2, &init_check_buffer[3]); TUSS4470_Read_Register(DEV_CTRL_3, &init_check_buffer[4]); TUSS4470_Read_Register(VDRV_CTRL, &init_check_buffer[5]); TUSS4470_Read_Register(ECHO_INT_CONFIG, &init_check_buffer[6]); TUSS4470_Read_Register(ZC_CONFIG, &init_check_buffer[7]); TUSS4470_Read_Register(BURST_PULSE, &init_check_buffer[8]); TUSS4470_Read_Register(TOF_CONFIG, &init_check_buffer[9]); TUSS4470_Read_Register(DEV_STAT, &init_check_buffer[10]); TUSS4470_Read_Register(DEVICE_ID, &init_check_buffer[11]); TUSS4470_Read_Register(REV_ID, &init_check_buffer[12]); } void TUSS4470_Read_Register(uint8_t regAddr, uint16_t *data) { uint8_t txbuffer[2] = {0}; // SPI transmit buffer uint8_t rxbuffer[2] = {0}; // SPI receive buffer txbuffer[0] = 0x80 + ((regAddr & 0x3F) << 1); // High byte: RW bit + address bits txbuffer[1] = 0x00; // Low byte: always 0 txbuffer[0] |= calculateParity(txbuffer); // Add parity bit TUSS4470_CSN_LOW(); // Pull chip select low HAL_Delay(500); HAL_SPI_TransmitReceive(&hspi1, txbuffer, rxbuffer, 2, HAL_MAX_DELAY); HAL_Delay(500); TUSS4470_CSN_HIGH(); // Pull chip select high *data = (rxbuffer[0] << 8) | rxbuffer[1]; // Combine received bytes } void TUSS4470_Write_Register(uint8_t regAddr, uint8_t data, uint16_t *write_back) { uint8_t txbuffer[2] = {0}; // SPI transmit buffer uint8_t rxbuffer[2] = {0}; // SPI receive buffer txbuffer[0] = (regAddr & 0x3F) << 1; // High byte: address bits txbuffer[1] = data; // Low byte: data txbuffer[0] |= calculateParity(txbuffer); // Add parity bit TUSS4470_CSN_LOW(); // Pull chip select low HAL_Delay(500); HAL_SPI_TransmitReceive(&hspi1, txbuffer, rxbuffer, 2, HAL_MAX_DELAY); HAL_Delay(500); TUSS4470_CSN_HIGH(); // Pull chip select high *write_back = (rxbuffer[0] << 8) | rxbuffer[1]; // Combine received bytes } static uint8_t calculateParity(uint8_t txbuffer[]) { uint8_t parity = 0; for (int i = 0; i < 2; i++) { uint8_t byte = txbuffer[i]; while (byte) { parity ^= (byte & 1); byte >>= 1; } } return parity; // Return calculated parity bit }
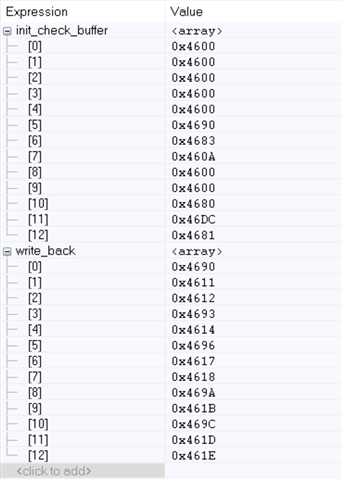
Thank you in advance for your help!